Migrating Objective-C Code to Swift
Learn how to migrate Objective-C code to Swift for cleaner, faster, and more stable applications with practical steps and best practices.
Essential Designs Team
|
April 23, 2025
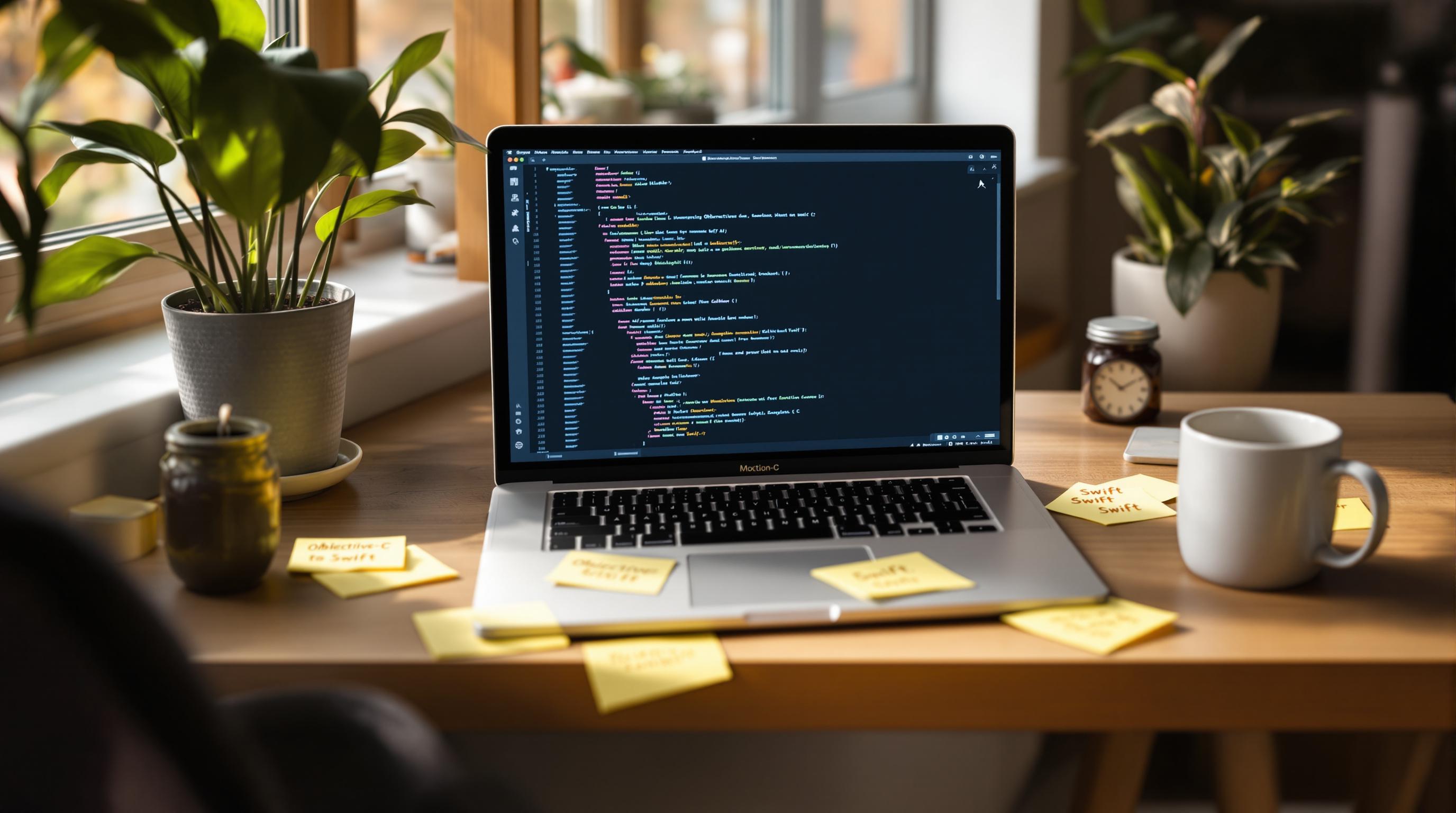
Migrating your Objective-C code to Swift can make your apps cleaner, faster, and more stable. Swift offers a modern syntax, fewer runtime errors, and better compatibility with Apple’s latest features. Here's how to get started:
- Benefits of Swift: Modern syntax, memory safety, faster builds, and better app performance.
- Preparation Steps: Clean up old Objective-C code, update syntax, and set up a bridging header for compatibility.
- Migration Options: Migrate file-by-file for large projects or convert the entire codebase for smaller ones.
- Testing: Write unit tests, ensure US-specific formats (dates, currency, etc.), and validate functionality.
Migrating Your App From Objective-C to Swift
Getting Your Code Ready
Before migrating to Swift, it's crucial to review and prepare your Objective-C codebase.
Clean Up Old Code
Start with a detailed review of your Objective-C code:
Remove Unused Code
Look for methods, classes, or dependencies that are no longer in use and remove them. Tools like Xcode's static analyzer can help pinpoint unreferenced code. A cleaner codebase makes migration smoother and reduces errors.
Update Syntax
Bring your code up to date by:
- Switching from manual memory management to Automatic Reference Counting (ARC)
- Changing old-style property declarations to modern dot notation
- Replacing deprecated API calls with their current versions
- Adding nullability annotations to improve compatibility with Swift
Simplify Complex Code
Break down lengthy or overly complex methods into smaller, easier-to-manage functions. This makes the migration process simpler and decreases the likelihood of runtime issues.
Project Setup Steps
Create a Bridging Header
Set up a bridging header to allow Objective-C and Swift to work together:
// ProjectName-Bridging-Header.h
#import "YourObjectiveCHeader.h"
Adjust Build Settings
In Xcode, update your Build Settings. Enable Defines Module
and Install Objective-C Compatibility Header
by setting them to YES. Also, specify the path to your bridging header.
Review and Update Dependencies
Go through your third-party libraries:
- Look for Swift versions of the Objective-C frameworks you're using
- Update your CocoaPods or Carthage configurations to include Swift-compatible versions
- Note any dependencies that may require special treatment during the migration
Set Up Testing
Write unit and UI tests for critical workflows. Integrate Continuous Integration (CI) to quickly identify and resolve issues.
Once your code is cleaned and your project is configured, you're ready to move on to the migration process.
Migration Steps
Choose Your Update Approach
You have two main options for updating your code:
- File-by-File Migration: Convert individual files or components step by step while keeping the application functional. This approach works well for larger codebases where changes can be isolated to specific modules.
- Complete Codebase Conversion: Rewrite the entire codebase in Swift. This is a better fit for smaller projects or when you have a dedicated team ready to tackle the migration all at once.
Enable Objective-C and Swift Interoperability
Expose Swift Code to Objective-C
To make your Swift code accessible in Objective-C files, import the Swift-generated header:
#import "YourModuleName-Swift.h"
Manage Common Type Mappings
For seamless compatibility, use @objc
annotations on classes or members that need to be shared between Swift and Objective-C. Also, ensure proper type conversions, such as mapping NSDictionary
to Dictionary
and NSArray
to Array
.
Finally, confirm everything is working by building the app and running both unit and UI tests.
sbb-itb-aa1ee74
Problems and Fixes
US Format Requirements
Use Foundation APIs to ensure locale-specific formatting for dates, numbers, currency, and measurements in en_US. Apply these adjustments during the final validation step.
// Date: MM/dd/yyyy
let df = DateFormatter()
df.locale = Locale(identifier: "en_US")
df.dateFormat = "MM/dd/yyyy"
let formattedDate = df.string(from: Date())
// Currency: $1,234.56
let nf = NumberFormatter()
nf.locale = Locale(identifier: "en_US")
nf.numberStyle = .currency
let formattedPrice = nf.string(from: 1234.56 as NSNumber)
// Decimal: 1,234.56
nf.numberStyle = .decimal
let formattedValue = nf.string(from: 1234.56 as NSNumber)
// Distance and temperature in US units
let distance = Measurement(value: 5.5, unit: .miles) // 5.5 mi
let temperature = Measurement(value: 75, unit: .fahrenheit) // 75 °F
After Migration Tasks
Once your code is running in Swift and you've confirmed everything works with US formats, it's time to wrap up the migration with regular reviews and updates.
Code Checks and Updates
Expand on your validation efforts by keeping track of any bugs using an issue tracker. Log defects found during unit and UI testing, and address them during your sprint cycles. This approach ensures problems are resolved efficiently. For comprehensive Swift migration support, from planning to deployment, consider teaming up with Essential Designs.
Using Modern Development Workflows
Set up automated testing pipelines and adopt continuous integration practices to maintain high-quality code. Take advantage of Swift's built-in tools and debugging features to monitor app performance and identify issues early in the development process.
Summary
A successful migration relies on careful planning, gradual implementation, and ongoing testing. Start by preparing your codebase and setting up Swift interoperability.
Move forward module by module, ensuring U.S. date and currency formats are accurate, and test thoroughly at every step.
Keep your code in top shape by following Swift best practices, conducting code reviews, and using continuous integration pipelines.
Leverage features like optionals, type inference, and protocol-oriented programming to improve safety, performance, and maintainability. This approach will help you make the most of Swift's capabilities.