Cross-Database Migration: Solving Data Type Conflicts
Learn how to tackle data type conflicts during cross-database migration to ensure data integrity and smooth operations.
Essential Designs Team
|
March 21, 2025
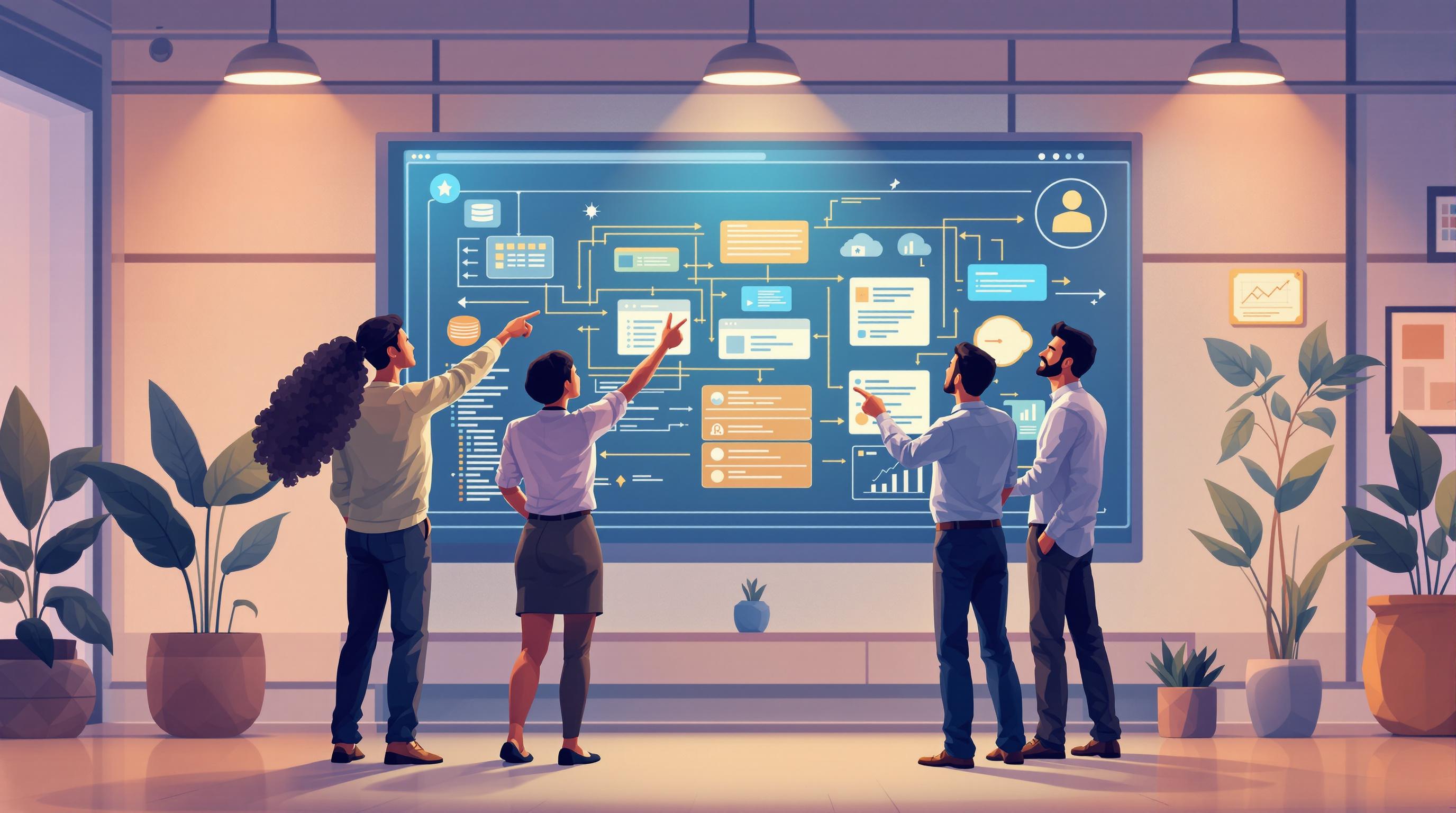
Cross-database migrations often face data type conflicts that can disrupt operations, cause errors, and compromise data integrity. Here's a quick guide to understanding and solving these challenges:
Common Issues:
- Numeric Data Conflicts: Differences in precision, range, or handling of types like
DECIMAL
,INTEGER
, andMONEY
. - Date/Time Inconsistencies: Variations in timestamp precision, time zone support, and date ranges across databases.
- Text Encoding Problems: Issues with character sets (e.g., UTF-8 vs. ASCII), string length limits, and multilingual support.
Solutions:
- Data Type Conversion: Map source data types to compatible target types using SQL transformations or tools.
- Null & Default Value Handling: Ensure consistent mapping of nulls, empty strings, and default values.
- Migration Tools: Use built-in tools like MySQL Workbench, pg_dump, or ETL software for automated type mapping.
- Custom Scripts: For complex cases, write custom SQL scripts to handle unique data type issues.
Best Practices:
- Schema Review: Compare source and target schemas, including data types, constraints, and indexes.
- Data Validation: Check for invalid ranges, orphaned keys, or encoding issues before migration.
- Testing: Conduct pre-migration, live, and post-migration tests for accuracy and consistency.
Quick Tip: Always document type mappings, validate data, and plan for rollbacks to avoid surprises during migration.
This article provides practical solutions, tools, and best practices to ensure a smooth transition between databases while preserving data accuracy and system functionality.
Full Convert Mapping Tables - Automatic and Manual Mapping
Main Data Type Conflicts Between Databases
When migrating data between databases, differences in numbers, dates, and text types often pose significant challenges.
Number Type Differences
Handling numeric data across databases can be tricky due to variations in precision and range. For example, MySQL's DECIMAL
type supports up to 65 digits, while SQL Server limits it to 38. These differences can lead to issues like data truncation or loss of precision during migration.
Here are some common conflicts:
- Integer Range Variations: PostgreSQL's
INTEGER
type ranges from –2,147,483,648 to +2,147,483,647. In contrast, Oracle'sNUMBER
type supports much larger ranges, which can cause overflow errors when working with big numbers. - Decimal Precision: MySQL's
FLOAT(4,2)
might store a value as 12.34, but Oracle'sNUMBER(4,2)
could round this differently. This inconsistency can be a problem, especially in financial calculations. - Currency Handling: SQL Server's
MONEY
type supports values up to $922,337,203,685,477.5807, while PostgreSQL applies different precision rules for itsMONEY
type. These differences can create issues during data transfers.
Date and Time Format Problems
Date and time formats often vary significantly across databases, creating challenges like:
- Timestamp Precision: Oracle supports up to nine decimal places for fractional seconds in timestamps, while MySQL only supports six. This can be problematic for applications that require microsecond-level precision.
- Time Zone Support: PostgreSQL's
TIMESTAMP WITH TIME ZONE
provides robust time zone handling, while MySQL'sTIMESTAMP
automatically converts values to UTC. This can lead to loss or misinterpretation of time zone data. - Date Ranges: SQL Server's
DATETIME
type supports dates starting from January 1, 1753, whereas MySQL'sDATETIME
begins from January 1, 1000.
Text Encoding Differences
Text data can also present challenges due to differences in encoding and field length limitations:
- Character Set and Length Compatibility: Migrating between systems with different character sets (e.g., UTF-8 vs. ASCII) can lead to encoding issues, such as corrupted special characters or emojis. Field length restrictions may further complicate this process.
- String Length Variations: MySQL's
VARCHAR
fields can hold up to 65,535 bytes, PostgreSQL supports strings up to 1 GB, and Oracle'sVARCHAR2
is limited to 4,000 bytes. - National Character Sets: Some databases use specialized Unicode types like Oracle's
NVARCHAR2
or SQL Server'sNVARCHAR
for multilingual text, while others handle it differently. These differences can cause compatibility issues during migration.
Addressing these conflicts is critical to ensuring data integrity when transitioning between systems. Properly resolving these discrepancies is a key step before diving into the conversion methods outlined in the next section.
How to Fix Data Type Conflicts
Data Type Conversion Methods
When handling data type conflicts, it's crucial to map source data types to compatible target types to ensure data integrity remains intact.
For numeric data, validate ranges before converting to avoid errors. Here's an example of converting a DECIMAL
to an INTEGER
with validation:
-- Example: Converting DECIMAL to INTEGER with validation
SELECT CASE WHEN source_decimal BETWEEN -2147483648 AND 2147483647
THEN CAST(source_decimal AS INTEGER)
ELSE NULL END AS converted_integer
FROM source_table;
For date and time values, standardizing formats can help maintain consistency. Use ISO 8601 format for clarity, as shown below:
-- Example: Standardizing datetime formats
SELECT CONVERT(VARCHAR(23), source_datetime, 126) AS standardized_datetime
FROM source_table;
Managing Null and Default Values
When dealing with null and default values, ensure consistent mapping between the source and target databases.
Here’s a quick guide:
Source Value | Target Handling | Rationale |
---|---|---|
NULL | NULL | Keep null values as they are |
Empty string | NULL or '' | Depends on target database semantics |
Default value | Equivalent default | Map to a matching default value |
To handle default text values, use a query like this:
-- Example: Default value handling
UPDATE target_table
SET text_column = COALESCE(source_column, target_default_value)
FROM source_table;
These practices help maintain consistency and avoid unexpected results during data type conversions.
sbb-itb-aa1ee74
Migration Tools and Software
Built-in Database Migration Tools
Many database systems come with built-in tools to handle type conflicts during migration. These tools are great for basic tasks like data type mapping and transformation:
Database System | Built-in Tool | Key Features |
---|---|---|
MySQL | MySQL Workbench | - Visual schema comparison and migration |
PostgreSQL | pg_dump/pg_restore | - Handles custom data types |
SQL Server | SQL Server Migration Assistant (SSMA) | - Automates type mapping |
Oracle | SQL Developer | - Supports cross-platform migrations |
To adjust type mappings before migration, you can use SQL commands like this:
-- Example: Custom type mapping in MySQL
ALTER TABLE target_table
MODIFY COLUMN numeric_column DECIMAL(10,2)
USING CAST(numeric_column AS DECIMAL(10,2));
For more intricate migrations, ETL tools might be a better fit.
ETL Software Options
ETL (Extract, Transform, Load) software offers advanced solutions for resolving data type conflicts. These tools often include user-friendly interfaces and built-in validation to streamline the transformation process.
Key features of ETL tools:
- Automated type detection: Identifies source data types and suggests compatible mappings.
- Data validation rules: Ensures data remains accurate during type conversions.
- Error handling: Detects and manages exceptions during the transformation process.
Here’s an example of how you might validate data in an ETL process:
-- Example: Data validation in ETL process
SELECT
CASE
WHEN TRY_CONVERT(datetime2, source_date) IS NOT NULL
THEN TRY_CONVERT(datetime2, source_date)
ELSE NULL
END AS validated_date
FROM source_table;
If neither built-in tools nor ETL software meet your needs, custom scripts can provide the flexibility required for complex scenarios.
Custom Scripts for Special Cases
Custom scripts are ideal when dealing with:
- Complex transformations that require specific business logic.
- Migrating legacy systems with unusual or unsupported data types.
- Multi-step conversions that need intermediate validation.
Here’s an example of a custom script for handling complex type conversions:
-- Example: Custom type conversion with validation
CREATE PROCEDURE ConvertCustomTypes
@SourceTable NVARCHAR(128),
@TargetTable NVARCHAR(128)
AS
BEGIN
-- Validate source data
WITH ValidationCTE AS (
SELECT
column_name,
CASE
WHEN TRY_CAST(source_value AS target_type) IS NULL
THEN 0
ELSE 1
END AS is_valid
FROM @SourceTable
)
-- Perform conversion for valid records
INSERT INTO @TargetTable
SELECT *
FROM ValidationCTE
WHERE is_valid = 1;
END;
For any migration approach, make sure to document type mappings, log errors, plan for rollbacks, and test thoroughly to avoid surprises.
Database Migration Best Practices
To ensure your database migration goes smoothly and without errors, it's essential to follow established practices that build on solid conversion and validation methods.
Database Schema Review Steps
Before diving into the migration process, taking the time to review the database schema can help you avoid potential issues, such as data type conflicts. Start by creating a mapping document that outlines the source and target data types.
Here are the key steps to focus on:
-
Schema Comparison Analysis: Create a document that compares the schemas, including:
- Column names and data types
- Relationships between primary and foreign keys
- Default values and constraints
- Indexes and configurations
- Data Type Compatibility Check: Map out the data types between the source and target systems. For example:
Source Type | Target Type | Potential Issue | Resolution Strategy |
---|---|---|---|
VARCHAR(50) | NVARCHAR(50) | Character encoding | Add conversion functions |
DATETIME | TIMESTAMP | Timezone handling | Apply timezone offset |
DECIMAL(10,2) | NUMERIC(10,2) | Precision differences | Validate decimal places |
BIGINT | INT | Value range overflow | Verify max values |
Once the schema is reviewed and mapped, you can shift your focus to ensuring data quality.
Data Quality Checks
Validating the quality of your data before migration is key to maintaining data integrity. Concentrate on these areas:
- Source Data Validation: Use SQL queries to check for invalid data ranges. For instance:
-- Validate numeric data ranges
SELECT column_name, COUNT(*) as invalid_count
FROM source_table
WHERE numeric_column NOT BETWEEN min_value AND max_value
GROUP BY column_name
HAVING COUNT(*) > 0;
- Data Consistency Verification: Check for issues such as orphaned foreign keys:
-- Check for orphaned foreign keys
SELECT fk_column, COUNT(*) as orphaned_records
FROM child_table c
LEFT JOIN parent_table p ON c.fk_column = p.pk_column
WHERE p.pk_column IS NULL
GROUP BY fk_column;
Testing and Verification Process
A multi-stage testing process is essential to verify the success of your migration:
-
Pre-Migration Testing: In your test environment, confirm:
- Record counts match between the source and target
- Data type conversions are accurate
- Business logic is preserved
- Performance benchmarks are met
- Migration Execution Validation: Track progress and identify errors during the migration:
-- Track progress and errors
SELECT
migration_step,
records_processed,
error_count,
CAST(error_count * 100.0 / records_processed AS DECIMAL(5,2)) as error_rate
FROM migration_log
ORDER BY step_timestamp;
- Post-Migration Verification: Compare the source and target data to ensure consistency:
-- Compare source and target data
WITH comparison AS (
SELECT
checksum_agg(binary_checksum(*)) as checksum
FROM source_table
UNION ALL
SELECT
checksum_agg(binary_checksum(*)) as checksum
FROM target_table
)
SELECT
CASE
WHEN COUNT(DISTINCT checksum) = 1 THEN 'Match'
ELSE 'Mismatch'
END as validation_result
FROM comparison;
Conclusion
Solutions Overview
Addressing data type conflicts requires a blend of technical know-how and careful validation. By using schema mapping, data type conversion methods, and thorough testing, you can safeguard data integrity while avoiding unnecessary downtime or errors.
Key steps include comparing schemas with data type mappings, applying conversion functions for incompatible types, validating data, and conducting multi-stage testing. With these measures in place, you can ensure a smoother migration process.
What to Do Next
Develop a clear roadmap and collaborate with professionals to minimize risks. Essential Designs has a proven track record in handling complex database migrations.
"We've been engaged with Essential Designs for several years now and we've found that the value they deliver is significantly above everyone else that we deal with." - Rick Twaddle, SBA, Teck Resources
As highlighted earlier, a detailed schema review, accurate data conversion, and comprehensive testing are non-negotiable. To set yourself up for success:
- Start with a detailed analysis of your current database structure.
- Identify potential data type conflicts and document solutions.
- Develop a robust testing plan, including pre-migration validation.
- Define clear success criteria and processes for verification.
- For complex migrations, consider bringing in experienced professionals.
Successful database migrations hinge on meticulous planning and execution. By following established practices and leveraging expert support, you can achieve seamless migrations while maintaining data accuracy and system functionality.
"Essential Designs was able to create a cutting edge application that will save lives, they always say 'Anything can be done' and are definitely able to deliver on that promise." - Jeff Hardy, Founder, Lifeguard